3.4 テキストのアンチエイリアス
3.4.1 テキストの描画品質
Graphics クラスの SmoothingMode プロパティの設定では、テキストの描画に対する描画品質を設定することはできません。テキストの描画に対してアンチエイリアスを有効にするかどうかという設定は、TextRenderingHint プロパティから行います。
public TextRenderingHint TextRenderingHint { get; set; }
このプロパティに設定するのは System.Drawing.Text.TextRenderingHint 列挙体のいずれかのメンバです。
[Serializable] public enum TextRenderingHint
基本的な考え方は図形のアンチエイリアスと同じです。滑らかなテキストを描画したい場合は高品質で低速なモードを、速度を重視する場合は高速で低品質奈モードを選択します。この列挙体には次のようなメンバが定義されています。
メンバ | 意味 |
---|---|
AntiAlias | グリッドに合わせないでアンチエイリアス |
AntiAliasGridFit | グリッドに合わせてアンチエイリアス |
ClearTypeGridFit | 最高の品質設定で ClearType 機能を利用する |
SingleBitPerPixel | グリッドに合わせないでアンチエイリアスを行わない |
SingleBitPerPixelGridFit | グリッドに合わせ、アンチエイリアスを行わない |
SystemDefault | ユーザーがシステムで選択した、すべてのフォント スムージング設定を使用 |
ClearType は、Windows XPで新たに追加された、マイクロソフトが開発したフォントのアンチエイリアス技術です。液晶ディスプレイ向けのアンチエイリアス技術とされているので、通常はブラウン管ディスプレイで選択するべきではないとされています。また、ClearType はカラーディスプレイの特徴を利用しているため、通常のアンチエイリアスとは異なり単純なグレースケースによる補完ではありません。そのため、モノクロディスプレイで ClearType を指定しても意味は無く、通常のアンチエイリアスと同じような効果となってしまいます。
using System.Drawing; using System.Drawing.Text; using System.Windows.Forms; public class Test : Form { protected override void OnPaint(PaintEventArgs e) { base.OnPaint(e); String text = "この魂に憐れみを"; Font font = new Font(FontFamily.GenericSerif, 26); Brush brush = new SolidBrush(Color.Black); int y = 0; e.Graphics.TextRenderingHint = TextRenderingHint.SystemDefault; e.Graphics.DrawString(text, font, brush, 0, y); e.Graphics.TextRenderingHint = TextRenderingHint.SingleBitPerPixel; e.Graphics.DrawString(text, font, brush, 0, y += font.Height); e.Graphics.TextRenderingHint = TextRenderingHint.SingleBitPerPixelGridFit; e.Graphics.DrawString(text, font, brush, 0, y += font.Height); e.Graphics.TextRenderingHint = TextRenderingHint.AntiAlias; e.Graphics.DrawString(text, font, brush, 0, y += font.Height); e.Graphics.TextRenderingHint = TextRenderingHint.AntiAliasGridFit; e.Graphics.DrawString(text, font, brush, 0, y += font.Height); e.Graphics.TextRenderingHint = TextRenderingHint.ClearTypeGridFit; e.Graphics.DrawString(text, font, brush, 0, y += font.Height); } static void Main() { Application.Run(new Test()); } }
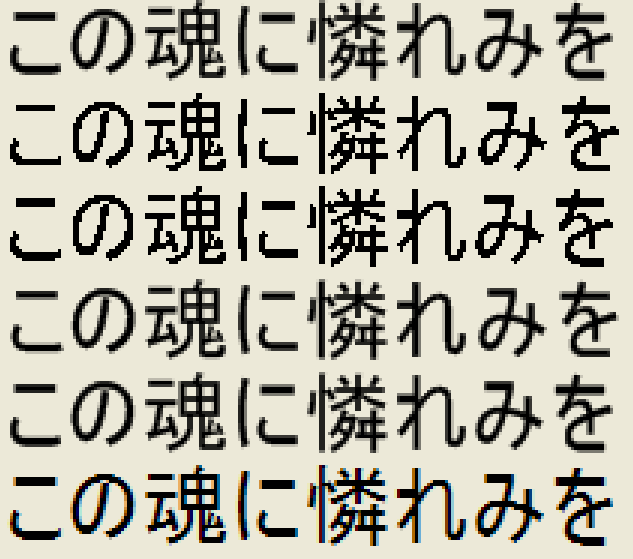
コード1は、それぞれのテキストレンダリング品質を TextRenderingHint 列挙体から設定して、同じテキストを描画しています。実行したウィンドウのスクリーンショットをビットマップとして保存し、これを拡大してみるとアンチエイリアスが行われているかどうかを確認できます。
アンチエイリアスを無効にした2段目と3段目のテキストは、見事に曲線部分にジャギが現れています。それに比べると、アンチエイリアスを有効にしたテキストはグレースケールで文字をぼかすようにジャギを補完しています。さらに、一番下の ClearType によるテキストの描画は、グレースケールではなくカラーでアンチエイリアスが行われます。